Your task for this project is to automate a login process in the following website:
https://the-internet.herokuapp.com/login
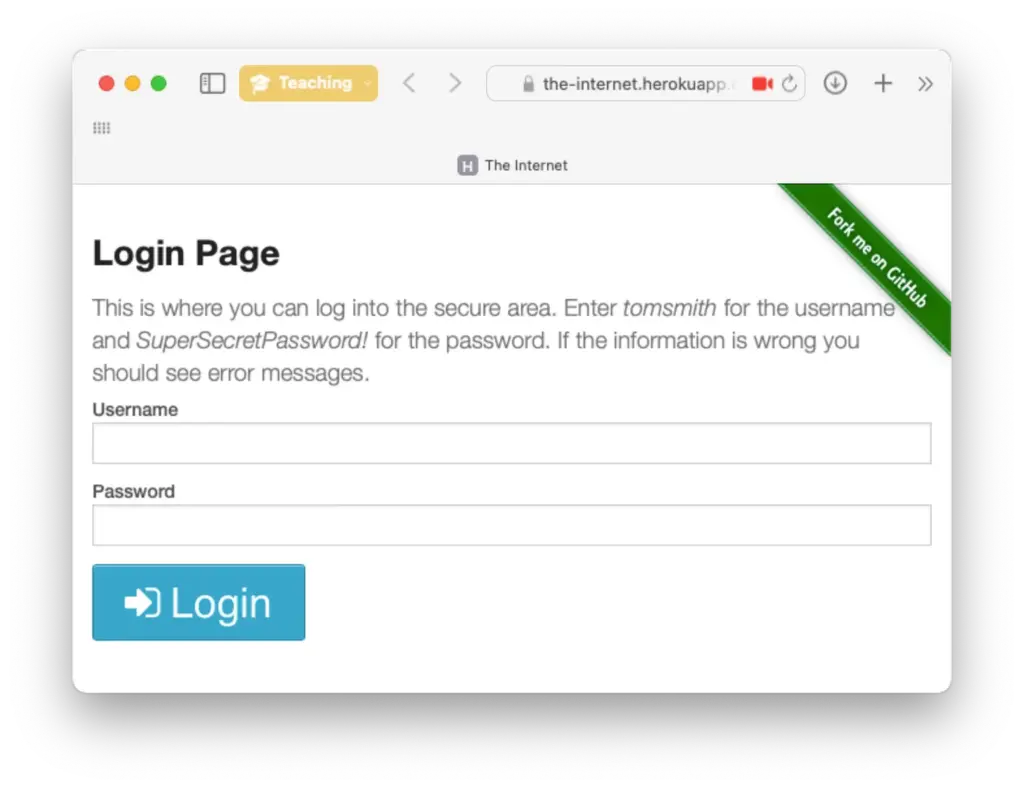
In other words, your Python script should:
- Start Chrome browser.
- Enter the login details in the Username and Password fields.
- Press the Login button.
- And finally display the logged in interface:
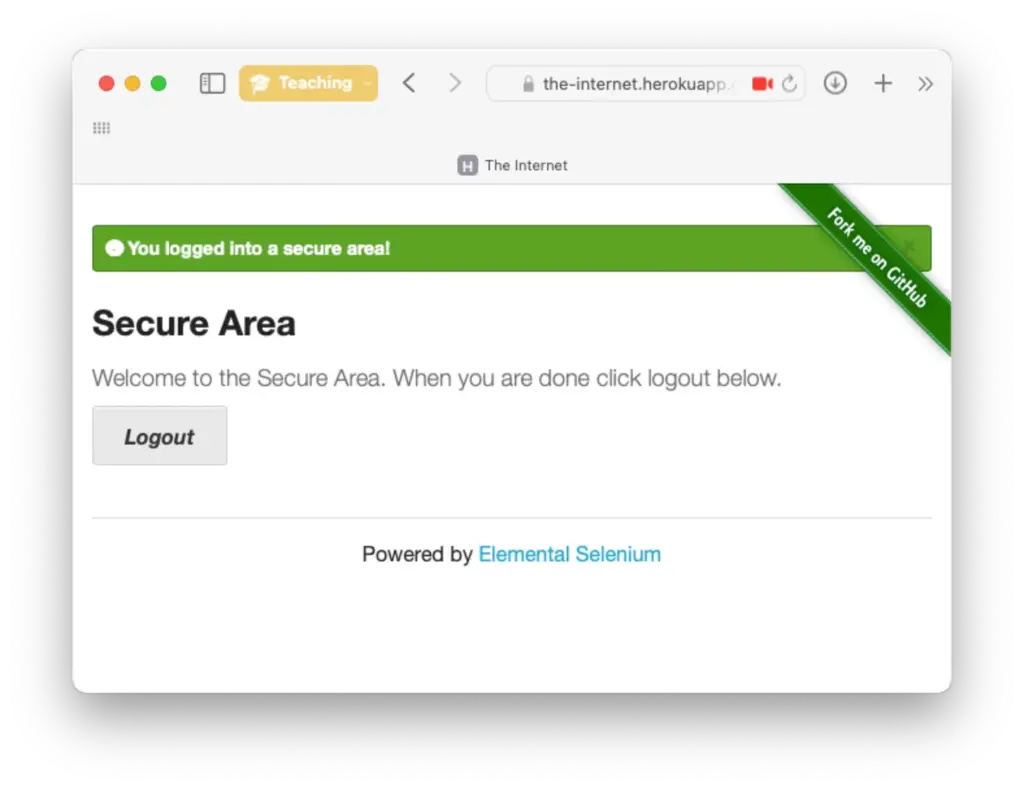
Use this username and password:
Username: tomsmith
Password: SuperSecretPassword!
Environment Setup Instructions
- Install the required libraries with:pip install selenium webdriver-manager
- Execute with python main.py and Chrome browser should open and you will automatically be logged in by Python.
Resources
Learn how to use Selenium to visit a webpage automatically with Python, do a search on the page, and click a link:
https://pythonhow.com/how/use-selenium-to-browse-a-page
Project Solution
main.py
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
import time
# Your login credentials
username = 'tomsmith'
password = 'SuperSecretPassword!'
# URL of the website you want to log in to
website_url = 'https://the-internet.herokuapp.com/login'
# Set up the Chrome WebDriver
service = Service(ChromeDriverManager().install())
driver = webdriver.Chrome(service=service)
# Open the website login page
driver.get(website_url)
# Locate the username and password fields and login button
username_field = driver.find_element(By.ID, 'username')
password_field = driver.find_element(By.ID, 'password')
login_button = driver.find_element(By.CSS_SELECTOR, 'button[type="submit"]')
# Enter your login credentials
username_field.send_keys(username)
password_field.send_keys(password)
# Click the login button
login_button.click()
# Optional: Wait for a few seconds to ensure the page loads completely
time.sleep(5)
# You can now perform other actions on the website as an authenticated user
# For example, you can get the page title to verify login success
print("Page title after login:", driver.title)
# Close the browser
driver.quit()
How to run the code
- Install the required libraries with:pip install selenium webdriver-manager
- Execute with python main.py and Chrome browser should open and you will automatically be logged in by Python.