Project Brief
This Flask app will let users enter a URL, and then it generates a QR code they can download as an image. Anyone who scans the generated QR code will be pointed to the URL associated with the generated QR code.
Expected Output
- The user can enter a URL and press the “Generate QR Code” button:
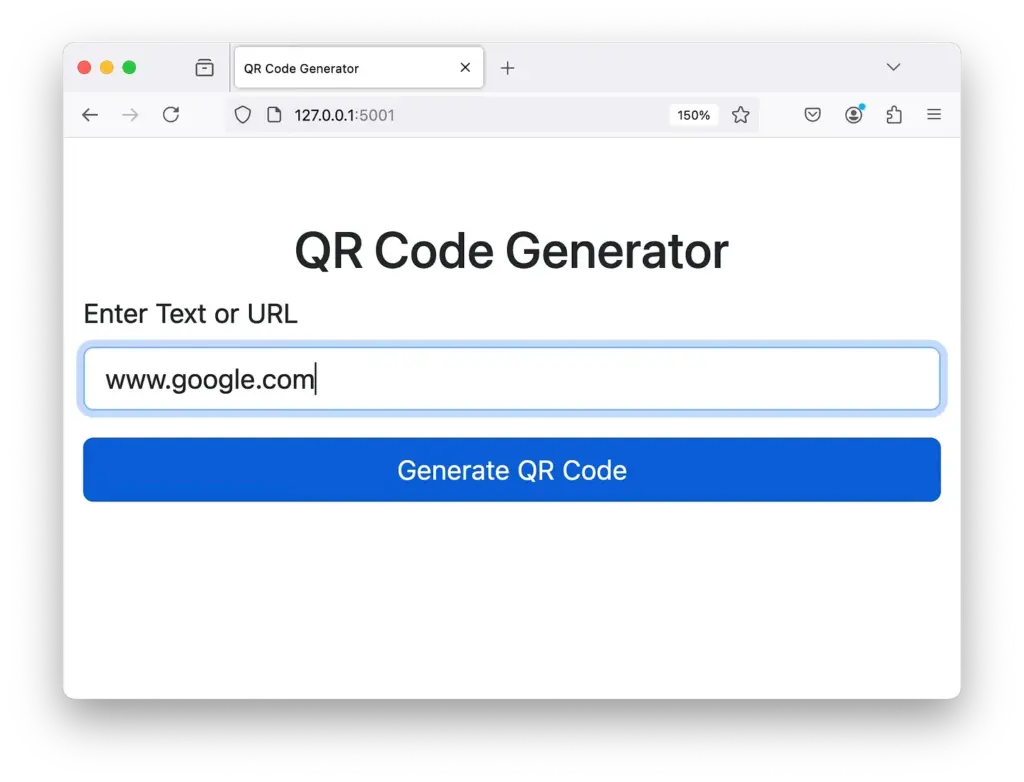
2. Once the user presses the button, a QR code PNG image is downloaded:
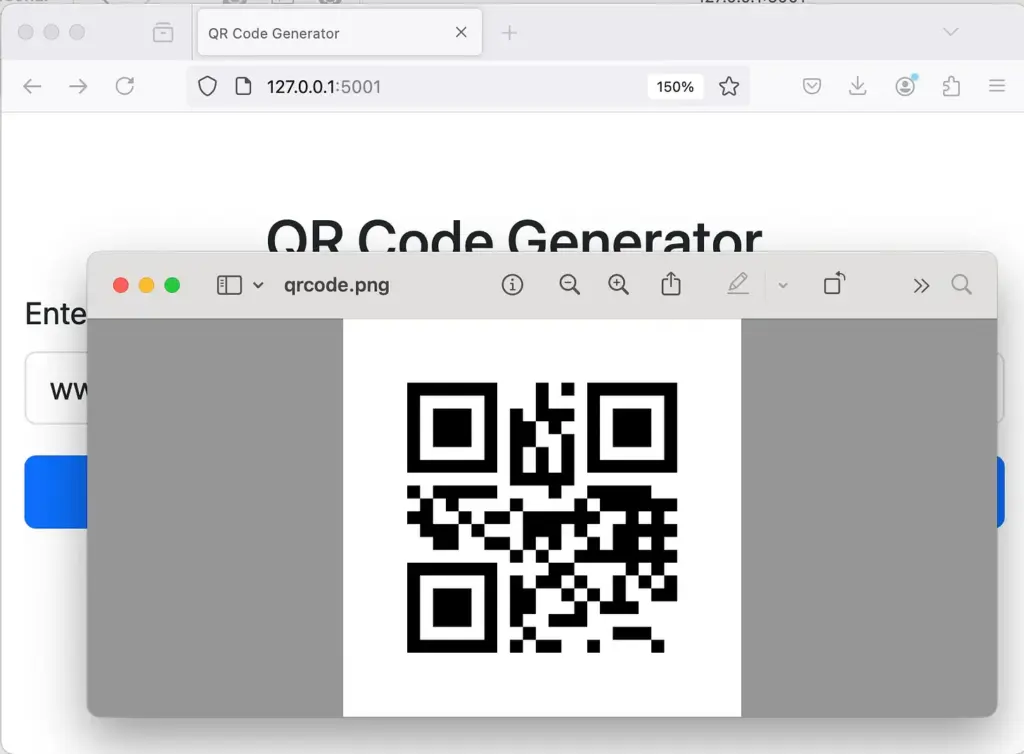
3. The user can scan the code with their mobile phone camera and the browser should open the page linked to the QR code:
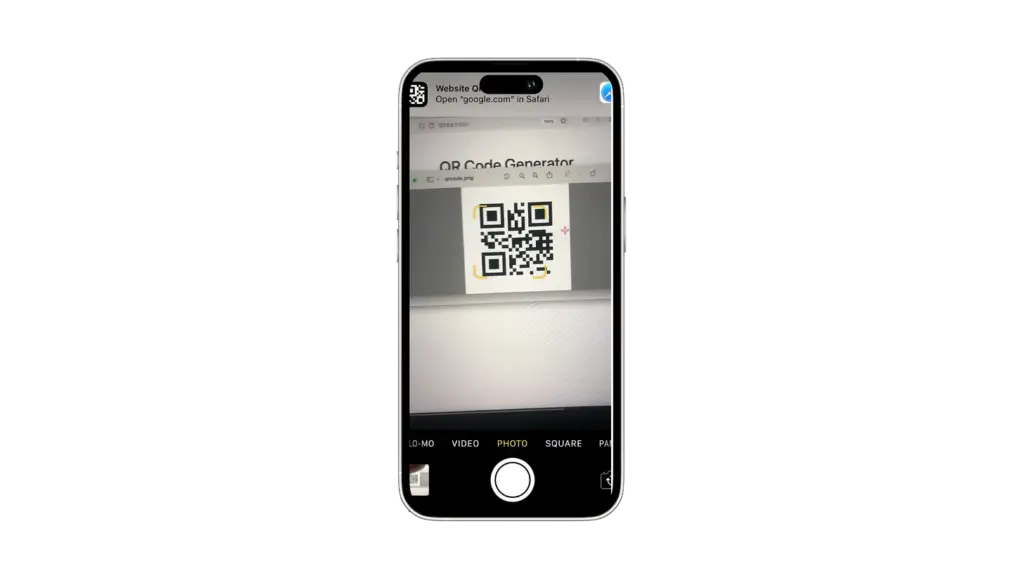
Prerequisites
Required Libraries: Flask, qrcode
pip install Flask qrcode
Required Files: No files are required for this project.
IDE: You can use any IDE on your computer to code the project.
Solution
Here is the code solution and the steps to get the app up and running:
- Create the proper file structure for your flask app:
app.py
templates/
index.html
2. Code for app.py:
from flask import Flask, render_template, request, send_file
import qrcode
from io import BytesIO
app = Flask(__name__)
@app.route('/', methods=['GET', 'POST'])
def index():
if request.method == 'POST':
text = request.form['text']
# Generate QR Code
qr = qrcode.QRCode(version=1, box_size=10, border=5)
qr.add_data(text)
qr.make(fit=True)
img = qr.make_image(fill='black', back_color='white')
# Save QR code to in-memory file
buffer = BytesIO()
img.save(buffer, 'PNG')
buffer.seek(0)
return send_file(buffer, mimetype='image/png', as_attachment=True, download_name='qrcode.png')
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
Code for index.html:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>QR Code Generator</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container">
<h1 class="mt-5 text-center">QR Code Generator</h1>
<div class="row justify-content-center">
<div class="col-md-6">
<form method="POST">
<div class="mb-3">
<label for="text" class="form-label">Enter Text or URL</label>
<input type="text" class="form-control" id="text" name="text" required>
</div>
<button type="submit" class="btn btn-primary w-100">Generate QR Code</button>
</form>
</div>
</div>
</div>
</body>
</html>
Run the app by executing app.py and then visit http://localhost:5000 in your browser to access the web app.